-
Minecraft Launcher
+
Launcher Settings
-
-
-
-
-
-
-
-
-
or
-
-
-
-
-
-
-
-
diff --git a/src/www/log_screen b/src/www/log_screen.html
similarity index 100%
rename from src/www/log_screen
rename to src/www/log_screen.html
diff --git a/src/www/logged_in b/src/www/logged_in.html
similarity index 100%
rename from src/www/logged_in
rename to src/www/logged_in.html
diff --git a/src/www/notification.html b/src/www/notification.html
new file mode 100644
index 0000000..99ebe0c
--- /dev/null
+++ b/src/www/notification.html
@@ -0,0 +1,42 @@
+
+
+
+
+
+
+
New message!
+
+
+
+ Lorem ipsum dolor sit amet consectetur adipisicing elit. Ipsam ea quo unde vel adipisci
+ blanditiis voluptates eum. Nam, cum minima?
+
+
+
+
+
\ No newline at end of file
diff --git a/src/www/portable.html b/src/www/portable.html
new file mode 100644
index 0000000..527b92b
--- /dev/null
+++ b/src/www/portable.html
@@ -0,0 +1,345 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ -
+
+
+
+ -
+
+
+
+ -
+
+
+
+ -
+
+
+
+
+
+
+
+
+
+
+
Welcome to Minecraft Launcher
+
Choose how you want to set up Minecraft.
+
+
+
+ Run in Portable Mode
+
+
No installation required. Saves everything in a local folder.
+
+
+
+ Install Minecraft
+
+
Installs Minecraft on your system for long-term use.
+
+
+
+
CraftX v1.0
+
+
+
+
+
+
+
+
+
+ Sign In
+
+
+
+
alterdekim
+
+
+
+
New instance
+
+
+
+
+
+
+
+ Download Version
+
+
+
+
or
+
+
+
+ Add MultiMC Instance
+
+
+
alterdekim
+
+
+
+
+
+
Account Settings
+
+
+
+
+
+
+
+
+
+
+
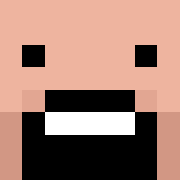
+
+
+ Upload
+
+
+
+
+
+
+
+
+

+
+
+ Upload
+
+
+
+
+
+
+ Save Settings
+
+
+
+
+
+
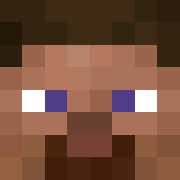
+
+
DartJevder
+ Blatnoe Pivo
+
+
+
+
+
+
+

+
+
Release
+ 1.12.2
+
+
+
+
+
+
+

+
+
Hypixel
+ mc.hypixel.net
+
+
+
+
+
+
+
+
+
+
\ No newline at end of file
diff --git a/src/www/profile_customization b/src/www/profile_customization.html
similarity index 100%
rename from src/www/profile_customization
rename to src/www/profile_customization.html
diff --git a/src/www/public_release b/src/www/public_release.html
similarity index 100%
rename from src/www/public_release
rename to src/www/public_release.html
diff --git a/src/www/sign_in.html b/src/www/sign_in.html
index 0f7cd15..f57bf5e 100644
--- a/src/www/sign_in.html
+++ b/src/www/sign_in.html
@@ -4,6 +4,7 @@
-
๐ Launcher Settings
+
+
Launcher Settings
-